How To Add Schools To Casper
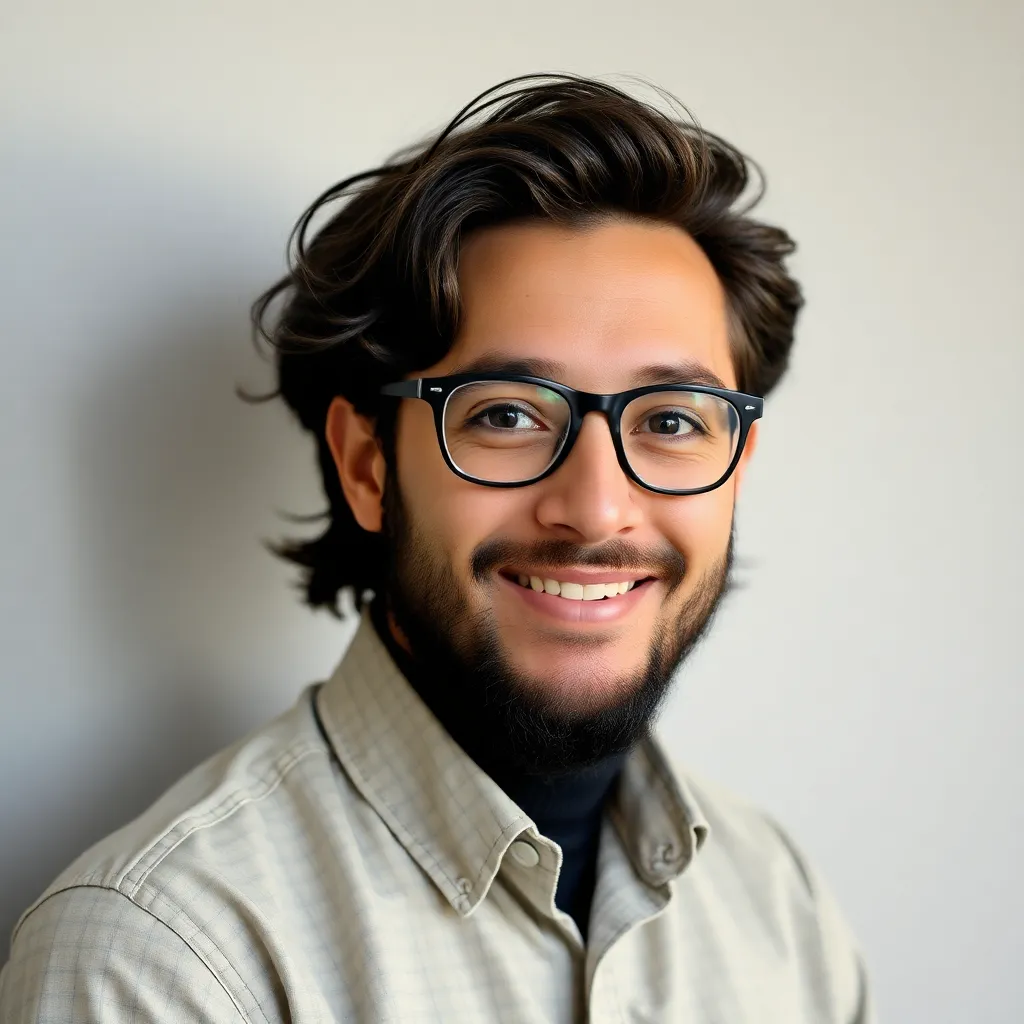
Ronan Farrow
Mar 17, 2025 · 3 min read

Table of Contents
How to Add Schools to CasperJS
CasperJS, a powerful navigation scripting & testing utility for PhantomJS, doesn't have a built-in function to directly add schools to a database or application. The process of "adding schools" depends entirely on the target application or system you're interacting with. This guide outlines strategies for common scenarios.
Understanding the Prerequisites
Before we delve into specific methods, it's crucial to understand the following:
- Target System: What system are you trying to add schools to? Is it a website with a form? An internal database accessed via an API? A CSV file? The approach varies drastically based on this.
- Data Source: Where is the school data coming from? A spreadsheet? An external API? Manual input? Having your data organized is essential.
- CasperJS Capabilities: CasperJS excels at automating web browser actions. For database interactions or file manipulation, you'll likely need to leverage other tools and libraries alongside it. This often means using Node.js modules within your CasperJS script.
Common Approaches & Code Examples
Let's explore common ways to interact with different systems:
1. Adding Schools via a Web Form
This is the most common scenario. If you're adding schools via a web form, CasperJS can automate the process:
casper.start('http://example.com/add-school', function() {
// Fill in the form fields
this.fill('form#school-form', {
'name': 'Example School',
'address': '123 Main Street',
'city': 'Anytown',
// ... other fields
}, true); // true submits the form
});
casper.run();
Explanation:
casper.start()
opens the URL of the form.this.fill()
populates the form fields with your data. Replace'form#school-form'
with the actual selector for your form and adjust the field names accordingly.true
submits the form.casper.run()
executes the script.
Important Considerations:
- Form Selectors: You need to inspect the form's HTML source to find the correct selectors for the form and input fields (e.g., ID, class name). Use your browser's developer tools to achieve this.
- Error Handling: The code lacks error handling. A robust script needs to check for successful submission and handle potential errors (e.g., network issues, invalid data).
- Captchas: If the website uses Captchas, you might need additional tools or services to overcome them; CasperJS alone cannot solve Captchas.
2. Interacting with an API
If the system uses an API, you'll need to use Node.js modules like request
or axios
to make API calls within your CasperJS script. This approach requires familiarity with the API's documentation. Here is a conceptual example:
var request = require('request');
casper.then(function() {
var schoolData = {
name: 'Example School',
address: '123 Main St'
// ...more data
};
request.post({
url: 'http://example.com/api/schools',
json: schoolData,
headers: {
'Content-Type': 'application/json'
}
}, function(error, response, body){
if(error){
this.echo("Error adding school: " + error, "ERROR");
} else {
this.echo("School added successfully");
}
});
});
casper.run();
Explanation: This snippet shows how to send a POST request to an API endpoint to add a new school. You would need to adapt it to your specific API's requirements, including the endpoint URL, authentication, and request body structure.
3. Working with CSV Files (Less Common)
While not a typical way to directly "add schools" to a system, if you have school data in a CSV file and need to process or pre-format it for input into a web form or API, you can use Node.js libraries like csv-parser
within your CasperJS script. This requires a separate step before interacting with the target system.
Remember to install necessary Node.js modules using npm install <module-name>
.
This guide provides a foundational understanding. The exact implementation depends heavily on the specific target system's interface and your data source. Always consult the relevant documentation and inspect the target system's structure for accurate selectors and API details. Prioritize error handling and security in your CasperJS scripts.
Featured Posts
Also read the following articles
Article Title | Date |
---|---|
How Thick Is Stone Veneer | Mar 17, 2025 |
How To Clean Acrylic Tank | Mar 17, 2025 |
How Often To Polish A Car | Mar 17, 2025 |
How Much Should You Tip A Party Bus Driver | Mar 17, 2025 |
How To Come Down From A Cocaine High | Mar 17, 2025 |
Latest Posts
Thank you for visiting our website which covers about How To Add Schools To Casper . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.