How To Fix Coupling Contractions
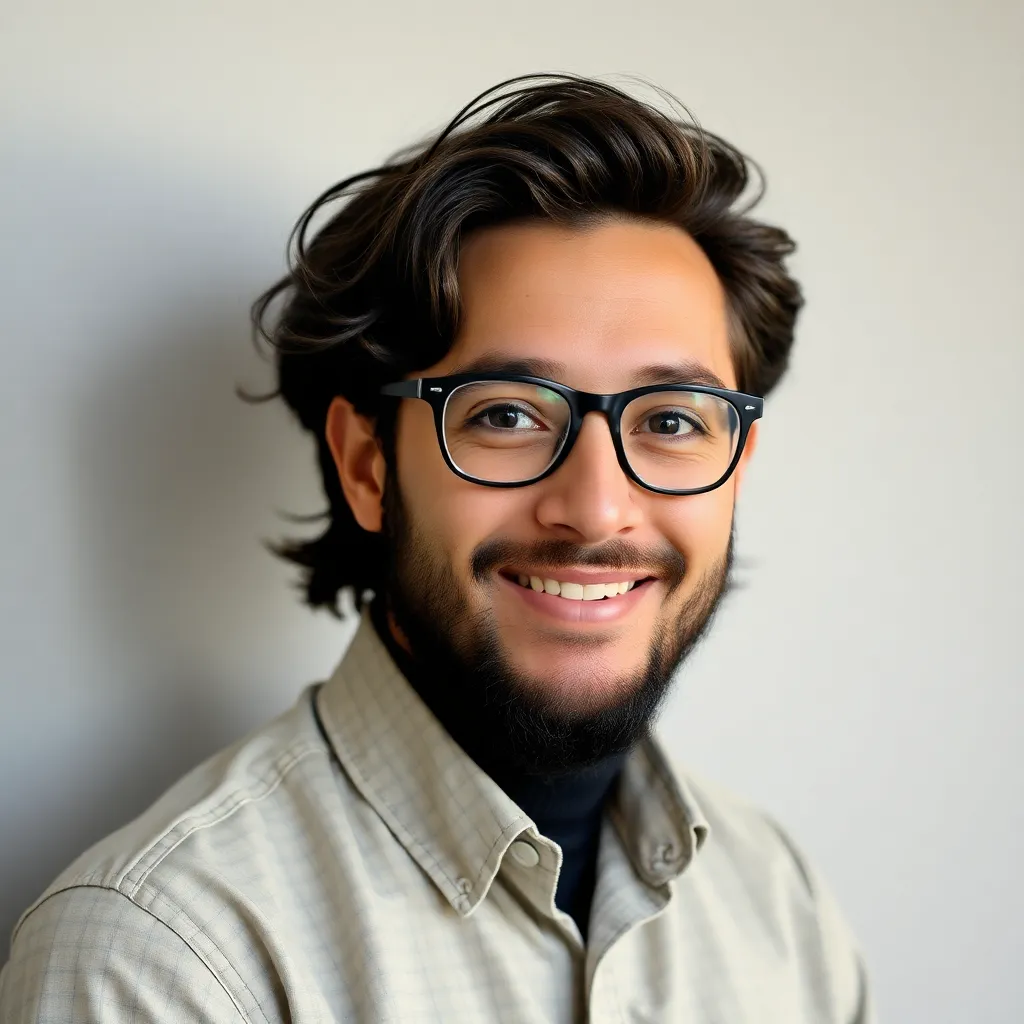
Ronan Farrow
Mar 18, 2025 · 3 min read

Table of Contents
How to Fix Coupling Contractions in Your Code
Coupling, in software engineering, refers to how closely different parts of a system are related. Tight coupling, where components are highly dependent on each other, can lead to brittle, hard-to-maintain code. Coupling contractions are essentially instances where this tight coupling manifests as problematic dependencies, making your code difficult to change, test, and reuse. This guide will explore how to identify and resolve these contractions.
Understanding Coupling Contractions
Before diving into solutions, it's crucial to understand what constitutes a coupling contraction. These aren't formal terms in the strictest sense, but rather a descriptive way of thinking about problematic dependencies. They usually manifest in these ways:
1. Direct Dependency on Concrete Implementations:
This is a classic example of tight coupling. Imagine a class that directly uses another class instead of an interface or abstract class. If the implementation of the used class changes, your dependent class will break.
Example (Bad):
class PaymentProcessor {
private PayPalPaymentGateway gateway = new PayPalPaymentGateway(); //Tight coupling
public void processPayment(double amount) {
gateway.processPayment(amount);
}
}
2. Excessive Method Parameters:
Passing numerous parameters to a method indicates a potential coupling problem. It suggests that the method is doing too much, and its dependencies are intertwined.
Example (Bad):
def sendEmail(to, subject, body, sender, server, port, username, password):
# ... Implementation ...
3. Shared Mutable State:
When multiple parts of your system rely on and modify the same mutable data, this creates a strong dependency. Changes in one area directly impact others, making it difficult to reason about the system's behavior.
Example (Bad):
let globalData = { count: 0 };
function increment() {
globalData.count++;
}
function decrement() {
globalData.count--;
}
Fixing Coupling Contractions: Strategies and Best Practices
The key to resolving coupling contractions is to reduce dependencies and improve modularity. Here are effective strategies:
1. Employ Dependency Inversion Principle:
This principle states that high-level modules should not depend on low-level modules. Both should depend on abstractions. Abstractions should not depend on details. Details should depend on abstractions. Use interfaces or abstract classes to define contracts, allowing for flexible implementation choices without affecting dependent modules.
Example (Good):
interface PaymentGateway {
void processPayment(double amount);
}
class PaymentProcessor {
private PaymentGateway gateway;
public PaymentProcessor(PaymentGateway gateway) {
this.gateway = gateway;
}
public void processPayment(double amount) {
gateway.processPayment(amount);
}
}
class PayPalPaymentGateway implements PaymentGateway { ... }
class StripePaymentGateway implements PaymentGateway { ... }
2. Refactor into Smaller, Focused Methods:
Break down large methods into smaller, more manageable units. This often leads to fewer parameters and clearer responsibilities.
Example (Good):
Instead of the sendEmail
example above, create separate methods for establishing a connection, composing the email, and sending it.
3. Introduce Facades or Mediators:
For complex interactions between multiple classes, create a facade that simplifies the interface and reduces direct dependencies. A mediator can be used to manage communication between objects, decoupling them.
4. Avoid Global State:
Limit the use of global variables and mutable shared state. Favor passing data explicitly between components or using immutable data structures.
5. Utilize Dependency Injection:
Dependency Injection frameworks provide a structured way to manage dependencies. This improves testability and maintainability. Instead of creating objects within a class, they are provided externally (through constructor injection, setter injection, or interface injection).
Conclusion
Addressing coupling contractions improves code quality, making it easier to maintain, extend, and test. By employing the strategies and best practices discussed above, you can create more robust and scalable software systems. Remember that refactoring is an iterative process. Start small, focusing on the most tightly coupled areas, and gradually improve the overall architecture of your codebase.
Featured Posts
Also read the following articles
Article Title | Date |
---|---|
How To Get On The Nice List | Mar 18, 2025 |
How To File For Separation In Illinois | Mar 18, 2025 |
How To Fix A Geek Bar | Mar 18, 2025 |
How To Fix Carbon Monoxide Leak In Furnace | Mar 18, 2025 |
How To Fix Leaks In Aluminum Boat | Mar 18, 2025 |
Latest Posts
Thank you for visiting our website which covers about How To Fix Coupling Contractions . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.