How To Unbind A Key In Rust
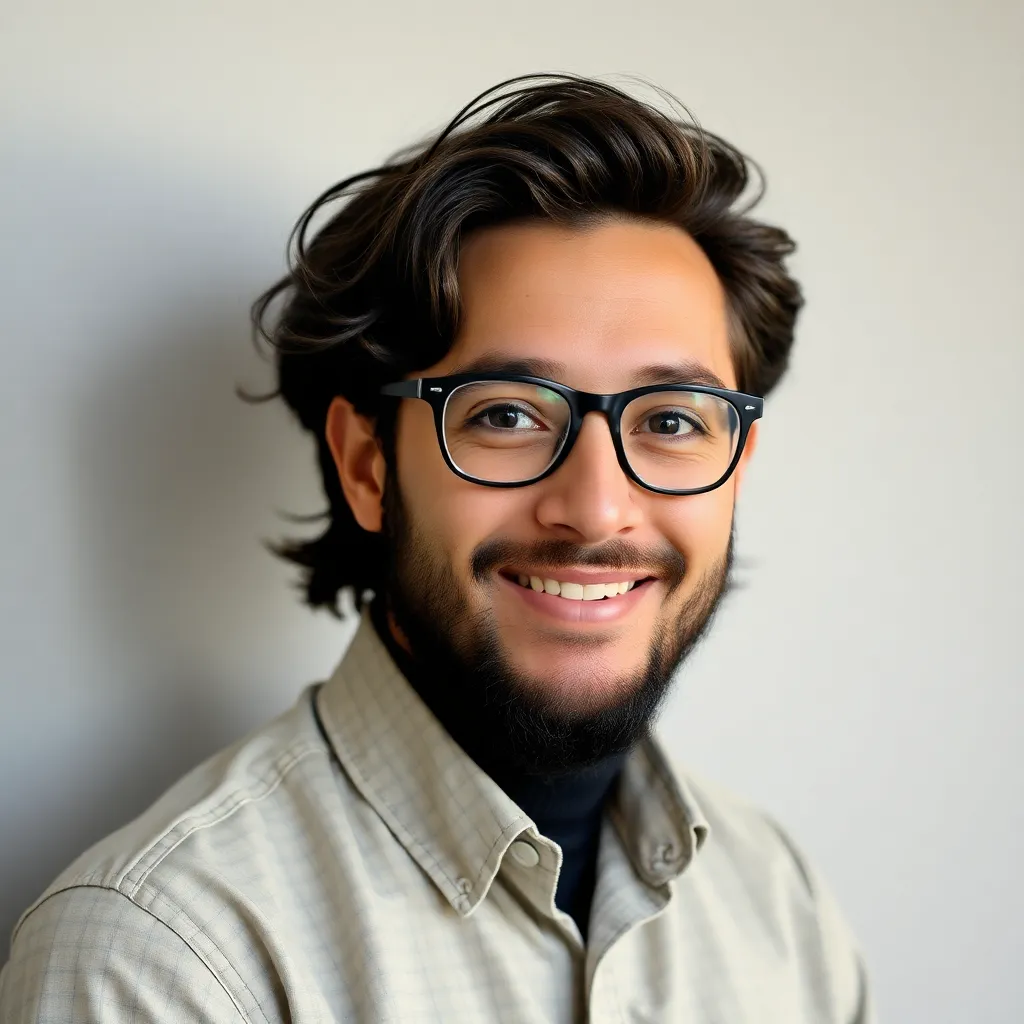
Ronan Farrow
Mar 20, 2025 · 3 min read
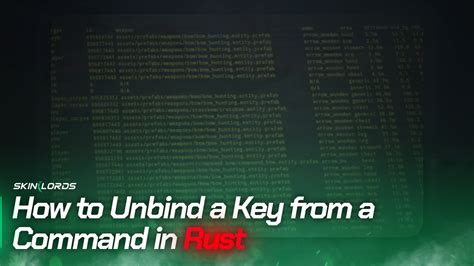
Table of Contents
How to Unbind a Key in Rust
This guide explains how to unbind or release a key in Rust, focusing on different contexts and scenarios you might encounter. Unbinding a key typically involves removing a key listener or event handler associated with a specific key. The exact method depends on the framework or library you're using for handling input.
Understanding Key Binding in Rust
Before we dive into unbinding, it's crucial to understand how keys are bound in the first place. Most Rust game development or GUI libraries work with event systems. When a key is pressed, an event is triggered. Your code registers a function (a callback) to handle these events. Unbinding involves removing this callback, preventing your code from responding to that specific key press.
Unbinding Keys in Different Contexts
The implementation differs significantly depending on the context:
1. Using a Game Development Library (e.g., ggez
, bevy
)
Game development libraries provide mechanisms for managing input. They often have functions specifically designed for removing key listeners.
Example (Conceptual):
Let's imagine a simplified example using a hypothetical game library:
// Hypothetical game library functions
fn bind_key(key: Key, callback: fn(KeyEvent)) { /* ... */ }
fn unbind_key(key: Key) { /* ... */ }
// ...in your game loop...
let jump_callback = |event: KeyEvent| { /* ... handle jump action ... */ };
bind_key(KeyCode::Space, jump_callback); // Bind spacebar to jump
//Later, to unbind:
unbind_key(KeyCode::Space); // Remove the jump action from the spacebar
Remember to replace this hypothetical code with the actual functions provided by your chosen game development library. Consult your library's documentation for precise instructions on key binding and unbinding. The specifics will vary significantly between ggez
, bevy
, and other libraries.
2. Using a GUI Library (e.g., iced
, egui
)
GUI libraries generally handle key events differently. They often operate based on widgets and their interactions, rather than directly managing global keybindings. You typically wouldn't "unbind" a key in the same way as a game; instead, you might disable or remove the widget that responds to the key press.
Example (Conceptual):
// Hypothetical GUI library
struct MyWidget {
enabled: bool, // ...
}
impl MyWidget {
fn update(&mut self, event: KeyEvent) {
if self.enabled && event.key == KeyCode::Enter {
// ...handle enter key press...
}
}
}
// ...in your application...
let mut my_widget = MyWidget { enabled: true };
// To "unbind", simply disable the widget:
my_widget.enabled = false;
This approach effectively prevents the widget from responding to the key press. Again, consult your chosen GUI library's documentation for specific instructions.
3. Operating System-Level Key Handling (Advanced)
Directly manipulating the operating system's keyboard input requires advanced techniques and is generally not recommended unless you are building a low-level application or driver. This typically involves using OS-specific APIs (Windows, macOS, Linux) which are beyond the scope of this basic tutorial.
Best Practices
- Always consult library documentation: The specific methods for key binding and unbinding are highly dependent on the library.
- Clear and concise code: Keep your key handling code organized and easy to understand.
- Error handling: Implement proper error handling to gracefully manage potential issues.
- Memory management: Be mindful of memory allocation and deallocation when working with callbacks.
This guide provides a general overview. The exact implementation of unbinding keys in Rust depends heavily on the specific libraries and frameworks you are using. Always refer to the official documentation of those libraries for the most accurate and up-to-date information.
Featured Posts
Also read the following articles
Article Title | Date |
---|---|
How Do Workers Compensation Attorneys Get Paid | Mar 20, 2025 |
How To Watch Movies On Uconnect While Driving | Mar 20, 2025 |
How To Test Hei Distributor | Mar 20, 2025 |
How You Doin Shirt | Mar 20, 2025 |
How Bad Does Tooth Extraction Hurt | Mar 20, 2025 |
Latest Posts
-
How Long Can An Air Compressor Run Continuously
Apr 05, 2025
-
How Long Can A Workers Comp Case Stay Open
Apr 05, 2025
-
How Long Can A Washing Machine Sit Unused
Apr 05, 2025
-
How Long Can A Tree Survive Out Of The Ground
Apr 05, 2025
-
How Long Can A Temporary Crown Stay On
Apr 05, 2025
Thank you for visiting our website which covers about How To Unbind A Key In Rust . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.