How To Use Onr
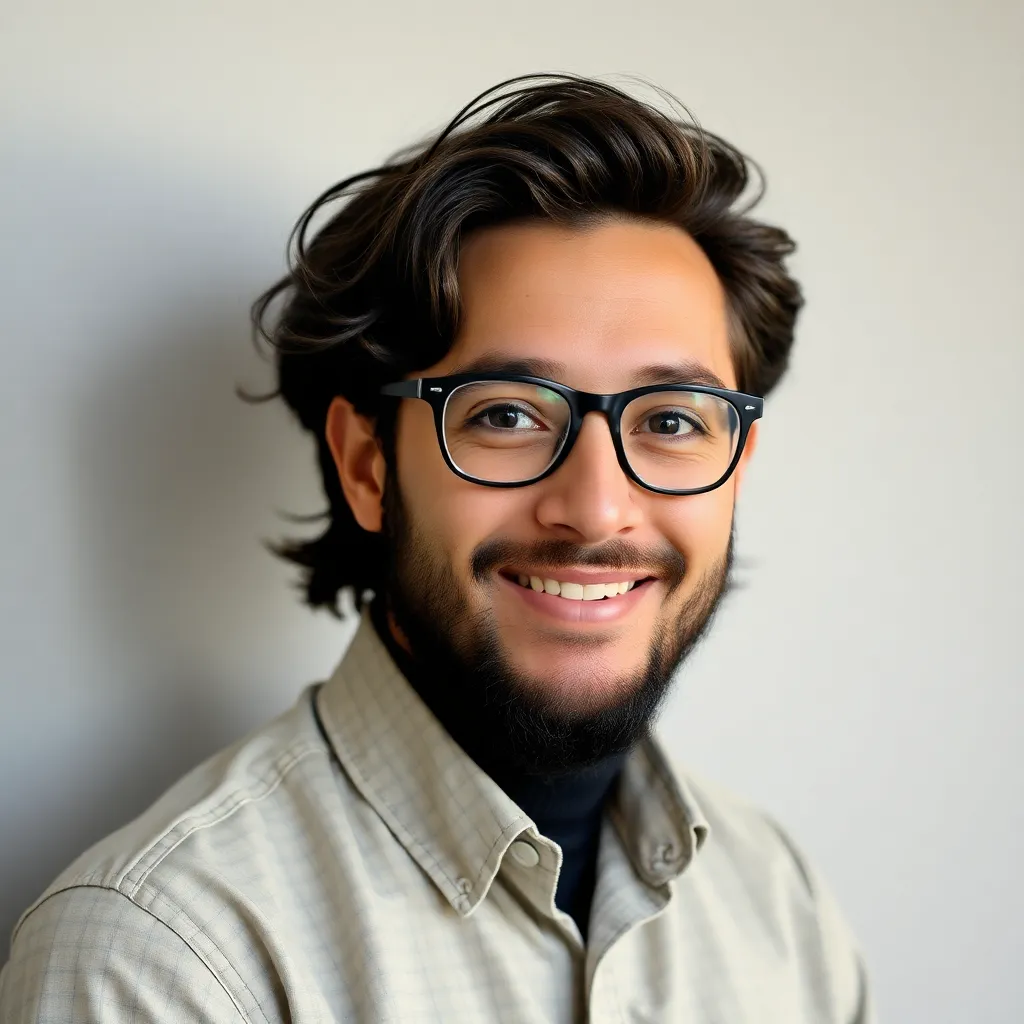
Ronan Farrow
Mar 20, 2025 · 3 min read
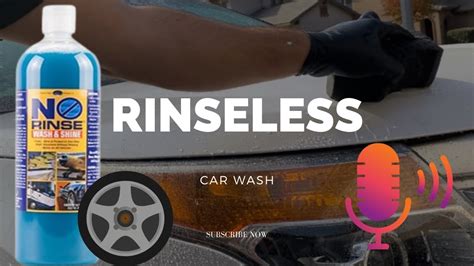
Table of Contents
How to Use ONR (Object-Oriented Navigation Robot) Effectively
ONR, or Object-Oriented Navigation Robot, isn't a widely recognized standardized term in robotics or programming. It's possible this refers to a specific internal project, a custom-built robot, or a less common acronym. However, the principles behind navigating using object-oriented programming principles can be applied to a wide range of robotics projects. This guide will assume you're building a robot that uses object-oriented principles for navigation, and provide advice on its effective use.
Understanding the Core Concepts
Before diving into specific implementations, let's review crucial concepts for object-oriented navigation:
1. Object Representation:
- Real-world objects: Identify the essential objects in your robot's environment (walls, obstacles, targets, etc.). Each will be represented as an object within your code.
- Object properties: Define properties for each object: position (x, y coordinates), size, shape, speed (if moving), etc. This data will drive your robot's decision-making.
- Data Structures: Choose appropriate data structures (e.g., classes, structs) to effectively store and manage this object information.
2. Object Interaction:
- Perception: Implement methods for your robot to 'perceive' its environment using sensors (e.g., lidar, cameras, ultrasonic). This perception informs the properties of the objects in your robot's world model.
- Navigation Logic: Create methods that describe how objects interact. For example, obstacle avoidance might be a method that takes the robot's current position and the positions of detected obstacles to calculate a new path.
- Path Planning: Implement algorithms (A*, Dijkstra's, etc.) to plan efficient paths around objects to reach the target location. This usually involves calculations based on object properties.
3. Encapsulation and Abstraction:
- Modular design: Organize your code into distinct modules (classes) responsible for specific aspects of navigation (e.g., a
SensorModule
for processing sensor data, aPathPlanningModule
for route calculation, aMotorControlModule
for executing movements). - Data hiding: Encapsulate data (object properties) within these modules, exposing only necessary interfaces (methods) for interaction. This improves code maintainability and reduces errors.
Practical Implementation Steps
Building a navigation system using object-oriented principles is iterative. Here's a suggested workflow:
1. Define Object Classes:
Create classes for different elements in your robot's environment. For instance:
// Example (Conceptual C++):
class Obstacle {
public:
double x, y;
double radius;
// ... other properties and methods ...
};
class Target {
public:
double x, y;
// ... other properties and methods ...
};
class Robot {
public:
double x, y;
// Methods for sensing, path planning, and motor control
};
2. Implement Sensor Integration:
Write code to receive data from your robot's sensors, and populate the properties of your object classes (obstacles, targets).
3. Develop Navigation Algorithms:
Implement path planning and obstacle avoidance algorithms. These algorithms should operate on the object data, ensuring efficient and safe navigation.
4. Motor Control:
Connect your navigation algorithms to the robot's motors to execute the planned paths.
5. Testing and Refinement:
Thoroughly test your system in a simulated environment and then on the real robot. Refine your algorithms and object representations based on the results.
Optimizing for Efficiency
For optimal performance:
- Data structures: Choose efficient data structures (e.g., spatial data structures like quadtrees or kd-trees) to speed up searching and path planning.
- Algorithm selection: Select suitable algorithms for your robot's capabilities and environment. A* is often preferred for its efficiency, but simpler algorithms may suffice in simpler environments.
- Real-time processing: Ensure your navigation system can process data and generate actions fast enough to keep up with the robot's movement.
This guide provides a framework for understanding and implementing object-oriented navigation. Remember that specific implementations will depend heavily on your robot's hardware, sensors, and the complexity of the environment it operates in. The key is to break down the navigation problem into manageable, well-defined objects and their interactions.
Featured Posts
Also read the following articles
Article Title | Date |
---|---|
How Do You Know If Your Water Pump Is Working | Mar 20, 2025 |
How Do You Make Liposomal Vitamin C | Mar 20, 2025 |
How Do You Know When God Wants You To Retire | Mar 20, 2025 |
How To Teach Place Value To First Graders | Mar 20, 2025 |
How To Wash Ksubi Jeans | Mar 20, 2025 |
Latest Posts
-
How Is Shaligram Formed
Apr 04, 2025
-
How Is Product Placement Different From Product Integration
Apr 04, 2025
-
How Is Praying In Jesus Name Sometimes Misinterpreted
Apr 04, 2025
-
How Is Cooper Remembered Today
Apr 04, 2025
-
How Is Community Coffee Decaffeinated
Apr 04, 2025
Thank you for visiting our website which covers about How To Use Onr . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.