How To View All Nodes Using Go Clinet
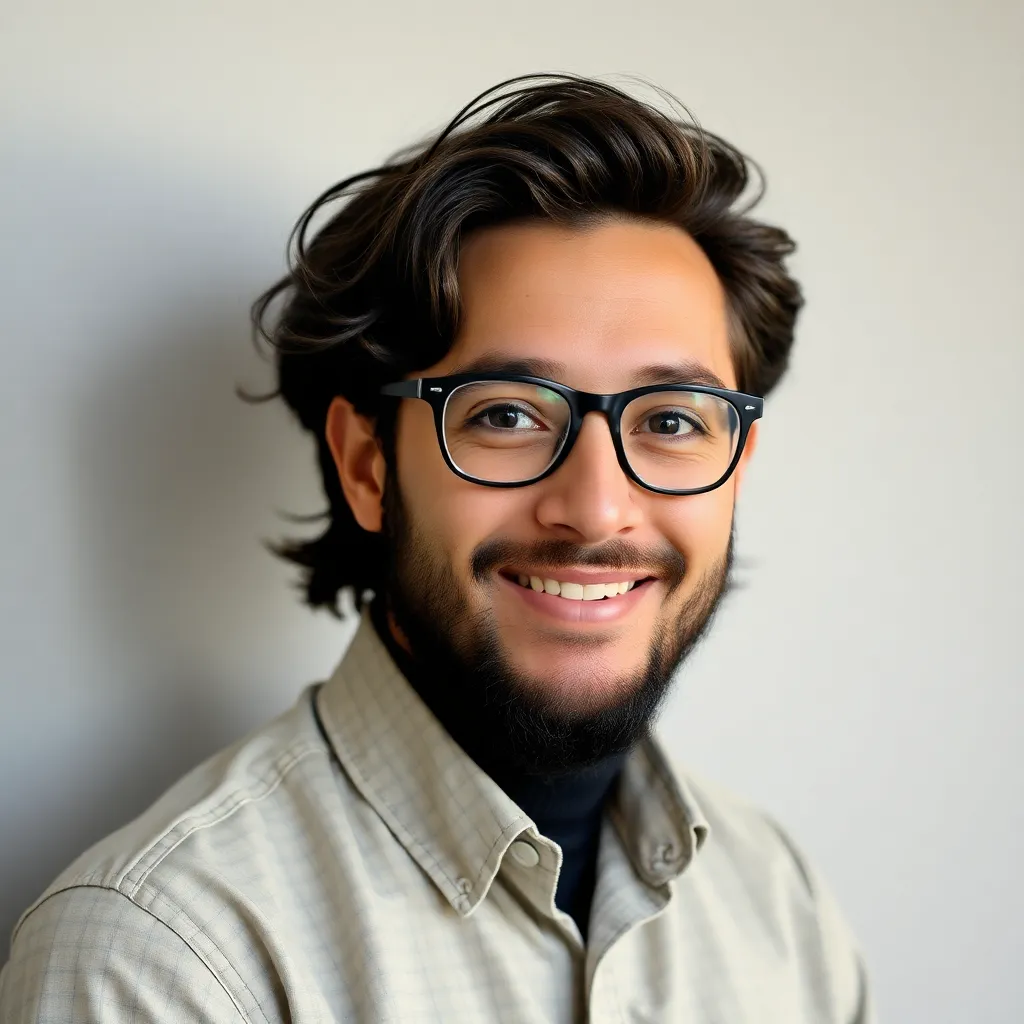
Ronan Farrow
Mar 03, 2025 · 3 min read

Table of Contents
How to View All Nodes Using a Go Client
This guide will walk you through the process of retrieving a list of all nodes within a network using a Go client. We'll focus on providing a clear, concise, and practical example, emphasizing best practices for readability and maintainability. Because the specifics heavily depend on the network's architecture and API, this will be a general approach adaptable to many situations. We'll assume you're working with a RESTful API.
Understanding the Prerequisites
Before we dive into the code, let's outline some crucial prerequisites:
- Network API Documentation: You absolutely need comprehensive documentation for the network's API. This documentation will specify the endpoints, request methods (GET, POST, etc.), and response formats (JSON, XML, etc.). It will also outline necessary authentication methods.
- Go Development Environment: Ensure you have Go installed and configured on your system. You'll need a suitable IDE or text editor.
- HTTP Client Library: Go's
net/http
package provides a basic HTTP client, but for more advanced features (like easier JSON handling), consider using a library likegithub.com/gorilla/mux
orgithub.com/go-resty/resty/v2
.
Implementing the Go Client
This example leverages the net/http
package for simplicity:
package main
import (
"context"
"encoding/json"
"fmt"
"net/http"
)
// Node represents the structure of a single node in the network. Adjust this to match your API's response.
type Node struct {
ID string `json:"id"`
Name string `json:"name"`
// Add other relevant fields here...
}
func main() {
// Replace with your network's API endpoint
apiEndpoint := "https://your-network-api.com/nodes"
// Create a new HTTP client with a context (for potential timeouts)
ctx := context.Background()
client := http.Client{}
// Create the request
req, err := http.NewRequestWithContext(ctx, http.MethodGet, apiEndpoint, nil)
if err != nil {
fmt.Println("Error creating request:", err)
return
}
// Add any necessary authentication headers here (e.g., API key)
// req.Header.Set("Authorization", "Bearer your_api_key")
// Send the request
resp, err := client.Do(req)
if err != nil {
fmt.Println("Error sending request:", err)
return
}
defer resp.Body.Close()
// Check for successful response
if resp.StatusCode != http.StatusOK {
fmt.Printf("Request failed with status code: %d\n", resp.StatusCode)
return
}
// Decode the JSON response into a slice of Nodes
var nodes []Node
if err := json.NewDecoder(resp.Body).Decode(&nodes); err != nil {
fmt.Println("Error decoding JSON:", err)
return
}
// Print the list of nodes
fmt.Println("Nodes:")
for _, node := range nodes {
fmt.Printf("ID: %s, Name: %s\n", node.ID, node.Name)
}
}
Remember to replace "https://your-network-api.com/nodes"
with the actual API endpoint for your node listing. Also, adjust the Node
struct to match the exact structure of the node data returned by your API. Error handling is crucial; the example includes basic checks, but you should implement more robust error handling in a production environment.
Handling Pagination (If Applicable)
Many APIs employ pagination to handle large datasets. If your network's API paginates results, you'll need to modify the code to handle multiple requests:
-
Check for Pagination Links: Examine the API response for links to subsequent pages. These links might be present in headers (
Link
header is common) or within the JSON response body. -
Iterate Through Pages: Create a loop that fetches each page until there are no more pages to retrieve.
-
Concatenate Results: Accumulate the nodes from each page into a single slice.
This will require a more sophisticated approach, potentially using recursion or a for
loop to handle the pagination mechanism dictated by your API.
Best Practices and Further Enhancements
- Error Handling: Implement more comprehensive error handling, including handling network errors, invalid JSON responses, and API-specific error codes.
- Context Management: Utilize context for managing timeouts and cancellation of requests.
- Rate Limiting: Incorporate mechanisms to handle rate limits imposed by the API.
- Logging: Add logging to track requests, responses, and errors.
- Testing: Write unit tests to ensure the correctness of your Go client.
This comprehensive guide provides a solid foundation for retrieving all nodes using a Go client. Remember to adapt it to your specific network's API and incorporate best practices for robust and maintainable code. Remember to always consult your network's API documentation for the most accurate and up-to-date information.
Featured Posts
Also read the following articles
Article Title | Date |
---|---|
How Long Does Divorce Take In Texas | Mar 03, 2025 |
How To Remove A Card Kinetic Hosting | Mar 03, 2025 |
How Wide Are Bmx Bars In The 80s In Inches | Mar 03, 2025 |
How To Transport A Shipping Container | Mar 03, 2025 |
How Long Do Apartment Tours Take | Mar 03, 2025 |
Latest Posts
Thank you for visiting our website which covers about How To View All Nodes Using Go Clinet . We hope the information provided has been useful to you. Feel free to contact us if you have any questions or need further assistance. See you next time and don't miss to bookmark.